ボタンでMatrix LEDを操作する
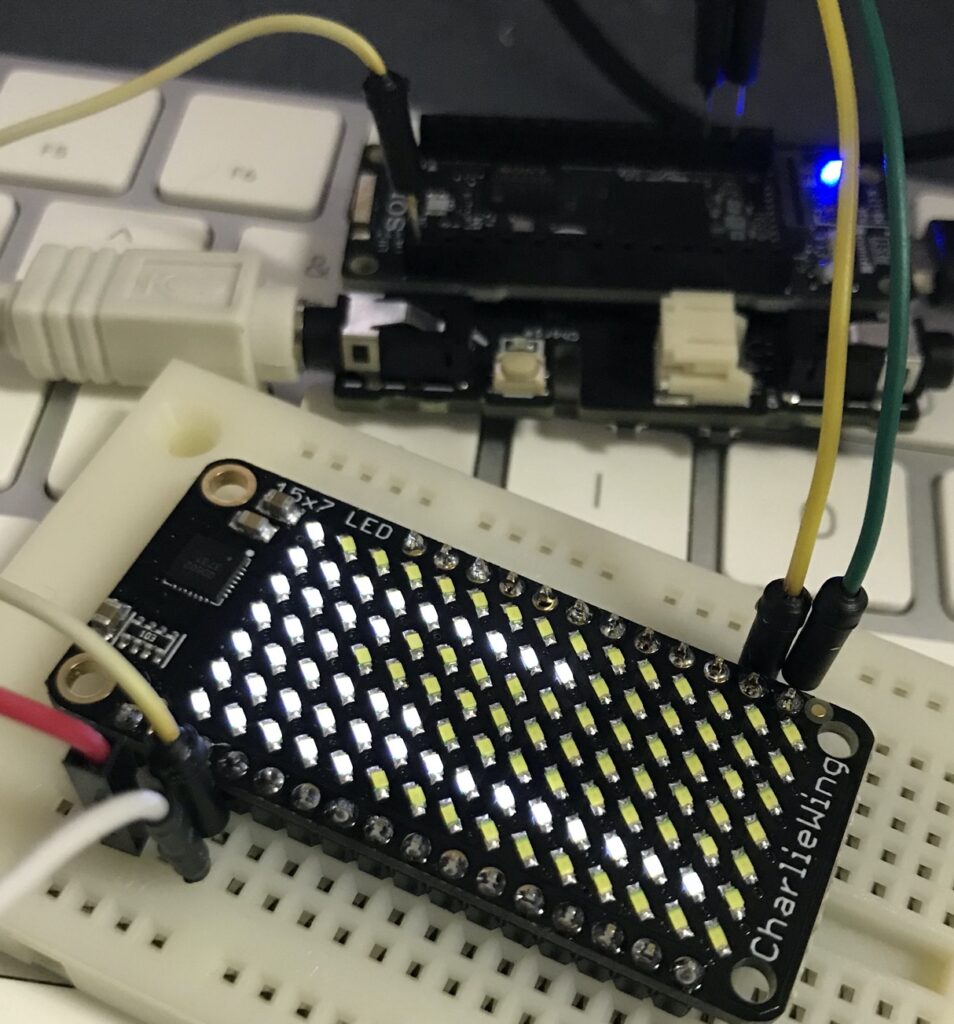
ボタンを押して、Matrix LEDを操作するプログラムを組んでみた。
これらを組み合わせて、7個のボタンを押すとLEDの表示が伸びるようにした。
プログラム
Matrix LEDの表示を attachInterrupt
のcallback関数内で変更すると、assertしてしまう。
まあ、そうだろうとわかるので、 loop()
の中で非同期に設定することにした。
また、ChangeState
フラフを用いて、 matrix.clear()
のによるちらつきを最小限にするように調整した。
#include <Wire.h>
#include <Adafruit_GFX.h>
#include <Adafruit_IS31FL3731.h>
// Initialize Matrix LED
Adafruit_IS31FL3731_Wing matrix = Adafruit_IS31FL3731_Wing();
// bars
#define WIDTH 7
// chanaged state
int8_t ChangeState = false;
static int8_t Bars[WIDTH];
void bar(int8_t x, int8_t y) {
Bars[x] += y;
ChangeState = true;
}
void IrqDSW0() {
Serial.print("DSW0\n");
bar(0, 1);
}
void IrqDSW1(){
Serial.print("DSW1\n");
bar(1, 1);
}
void IrqDSW2(){
Serial.print("DSW2\n");
bar(2, 1);
}
void IrqDSW3(){
Serial.print("DSW3\n");
bar(3, 1);
}
void IrqDSW4(){
Serial.print("DSW4\n");
bar(4, 1);
}
void IrqDSW5(){
Serial.print("DSW5\n");
bar(5, 1);
}
void IrqDSW6(){
Serial.print("DSW6\n");
bar(6, 1);
}
void attachIRQ() {
pinMode( PIN_D12, INPUT_PULLUP );
pinMode( PIN_D07, INPUT_PULLUP );
pinMode( PIN_D06, INPUT_PULLUP );
pinMode( PIN_D05, INPUT_PULLUP );
pinMode( PIN_D09, INPUT_PULLUP );
pinMode( PIN_D03, INPUT_PULLUP );
pinMode( PIN_D11, INPUT_PULLUP );
pinMode( PIN_D10, INPUT_PULLUP );
attachInterrupt( PIN_D12, IrqDSW0, FALLING );
attachInterrupt( PIN_D07, IrqDSW1, FALLING );
attachInterrupt( PIN_D06, IrqDSW2, FALLING );
attachInterrupt( PIN_D05, IrqDSW3, FALLING );
attachInterrupt( PIN_D09, IrqDSW4, FALLING );
attachInterrupt( PIN_D03, IrqDSW5, FALLING );
attachInterrupt( PIN_D11, IrqDSW6, FALLING );
}
void setup() {
Serial.begin(115200);
Serial.println("ISSI manual animation test");
if (! matrix.begin()) {
Serial.println("IS31 not found");
while (1);
}
Serial.println("IS31 Found!");
for (int8_t n = 0; n <= WIDTH; n++) {
Bars[n] = 0;
}
// set interrupt functions
attachIRQ();
}
void loop() {
if (ChangeState == false) {
// no change
return;
}
matrix.clear();
for (int8_t n = 0; n <= WIDTH; n++) {
matrix.drawLine(0, n, Bars[n], n, 127);
}
ChangeState = false;
delay(100);
}