QMKでArduino用キーボードを作る (1/4 テスター編)
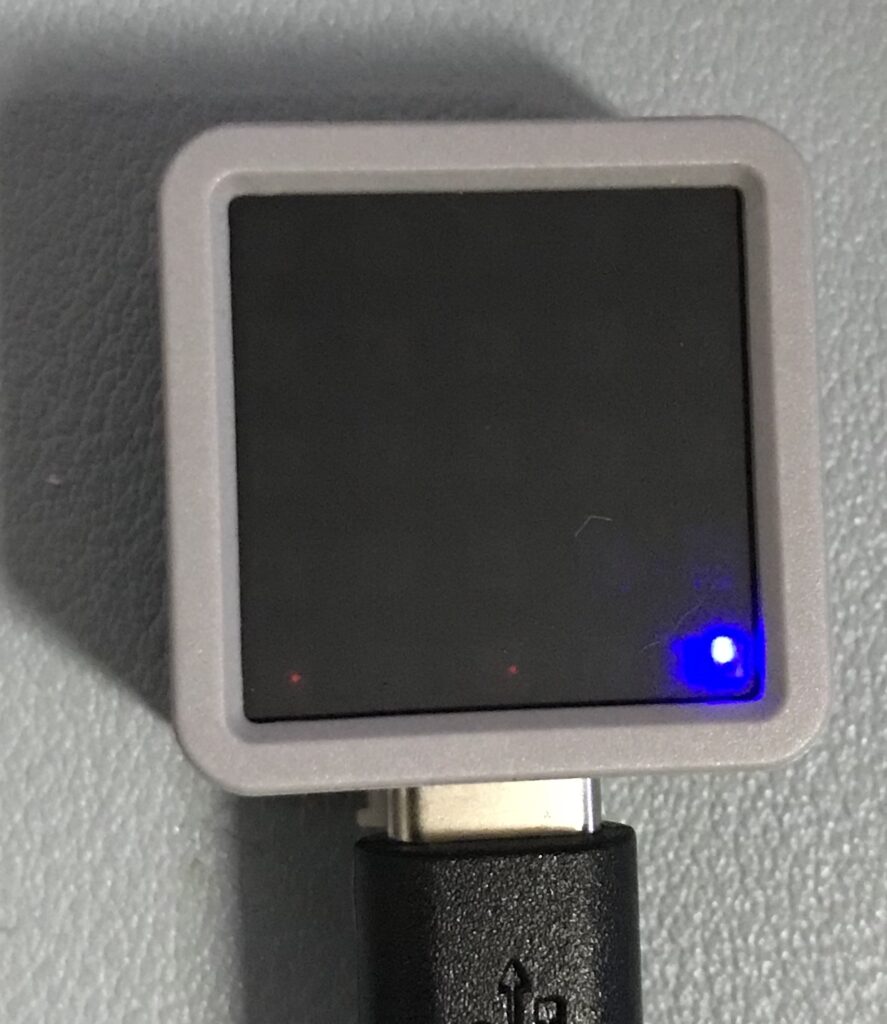
キーボード #2 Advent Calendar 2020
に参加しています。
3日目 Arduino
やりたいこと
Arduino用のキーボードを作ってみる。
正しくは、Arduino で利用できるキーボードをQMKで作る。
USB(HID)のキーボードは、 su120キットのおかげで作れるようになったので、それを改造する。
special thanks to @e3w2q さん
完成図
Arduino 側は、ATOM Matrix で作成。今後もテスターとして利用できるようにする。
通信はをシリアル接続(8N1)で、ATOM Matrixには押されたキーの位置をLEDで表示する。
ATOM Matrix は、ESP32 Pico に 5×5 のフルカラーLEDを実装したかわいいやつ。
I/F仕様
シリアル通信の仕様(Network層)
- 電圧 5V
- bitrate … QMXの最低である20Kbps
- bit数 … 8bits
- parity … なし
- stop bit … 1bit
キーのMatrixデータ仕様 (Transport層)
1回の送信で7bytes。
byte | bits | 値 | 説明 |
---|---|---|---|
1 | 1-5 | 0 or 1 | キーボード1行目の押し込み状態 |
2 | 1-5 | 0 or 1 | キーボード2行目の押し込み状態 |
3 | 1-5 | 0 or 1 | キーボード3行目の押し込み状態 |
4 | 1-5 | 0 or 1 | キーボード4行目の押し込み状態 |
5 | 1-8 | 0-127 | 左側のロータリーエンコーダーの値 |
6 | 1-8 | 0-127 | 右側のロータリーエンコーダーの値 |
7 | 1-8 | 0xff | データの最後EOF |
ex
押したキー | Matrixデータ |
---|---|
なし | 00:00:00:00:00:00:ff |
1行目1列 | 80:00:00:00:00:00:ff |
2行目1列 | 00:80:00:00:00:00:ff |
2行目1列と2列 | 00:c0:00:00:00:00:ff |
2列目全部 | 40:40:40:40:00:00:ff |
3行目のキー5個全部 | 00:00:f8:00:00:00:ff |
ATOM Matrix でテスターを作成
QMK の改造をする前に、正しい動作を確認するためのテスターを作る。
シリアル通信部分
だれでも簡単。Serial のread でデータを読める。
例えば、こんな感じ。
void setup() {
...
// Serial1 の設定
Serial1.begin(20000, SERIAL_8N1, PIN_RX, PIN_TX);
}
void loop() {
if (Serial1.available()) {
int inByte = Serial1.read()
if (inByte == 0xff) {
// stop data
テスターの実装
テスター仕様は、ATOM Matrix の5×5のLEDのうち、上位4行でキーボードの押した位置を点灯。
5行目の1,2カラムは、左のエンコーダ値。
5行目の3,4カラムは、右のエンコーダ値。
5行目の5カラムは、serialでデータを受け取ると、点滅する。
全部のキーを押すとこんな表示になる。